Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- visualstudio2022
- 3차원배열
- uidesign
- 배열문제
- 렌더몽키
- c++class
- 게임만들기
- premake5
- 화살피하기
- 화살표 메서드
- 공부
- 표창던지기
- 언리얼
- Unity
- 이득우
- swipe
- 이득우언리얼
- python
- 셰이더
- IMGUI
- 파이썬
- C++
- 그림자 효과
- 비주얼스튜디오
- 유니티
- 다중상속
- 화살표 함수
- rendermonkey
- c#
- 가변배열
Archives
- Today
- Total
신입 개발자 공부 과정
01/06 과제 본문
Q1.해당 내용을 엑셀 내용으로 다음과 같이 작성해본다.
1. JSON파일을만든다
2. JSON파일을 읽는다
3. 맵핑클래스를 만든다
4. 읽어온 json 문자열을 역직렬화 한다
5. 사전에 넣는다
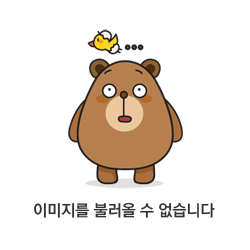
Json 뷰어에서의 모습
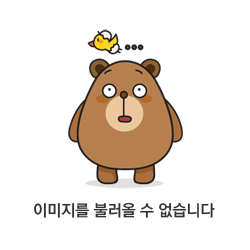
Json text 형식
[
{
"id": 500,
"name": "레저릭의 호기",
"level": 41,
"goal": "소금 평원에 있는 래저릭에게 시포리움 부스터를 찾아다 줘야 합니다."
},
{
"id": 501,
"name": "안전제일",
"level": 41,
"goal": "가젯잔에 있는 쉬리브에게 시포리움 부스터를 가져가야 합니다."
},
{
"id": 502,
"name": "일어나라, 흑요암이여!",
"level": 46,
"goal": "검은 라소릭과 흑요암을 쓰러뜨리고, 그 증거로 검은 라소릭의 머리카락과 흑요암 심장을 아이언포지에 있는 소리우스에게 가져가야 합니다."
}
]
1. JSON파일을만든다
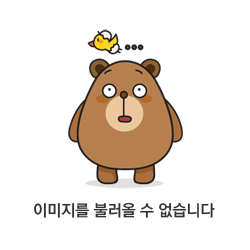

2. JSON파일을 읽는다
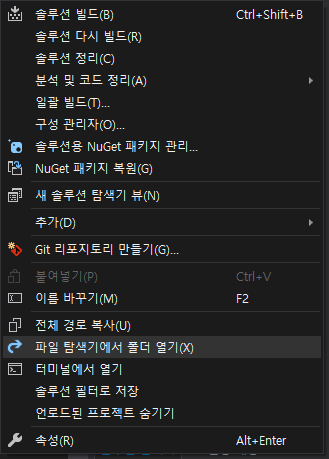
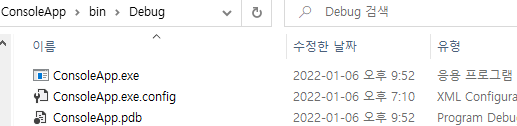
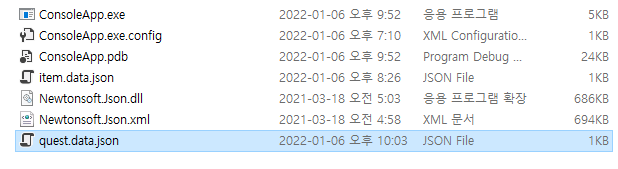
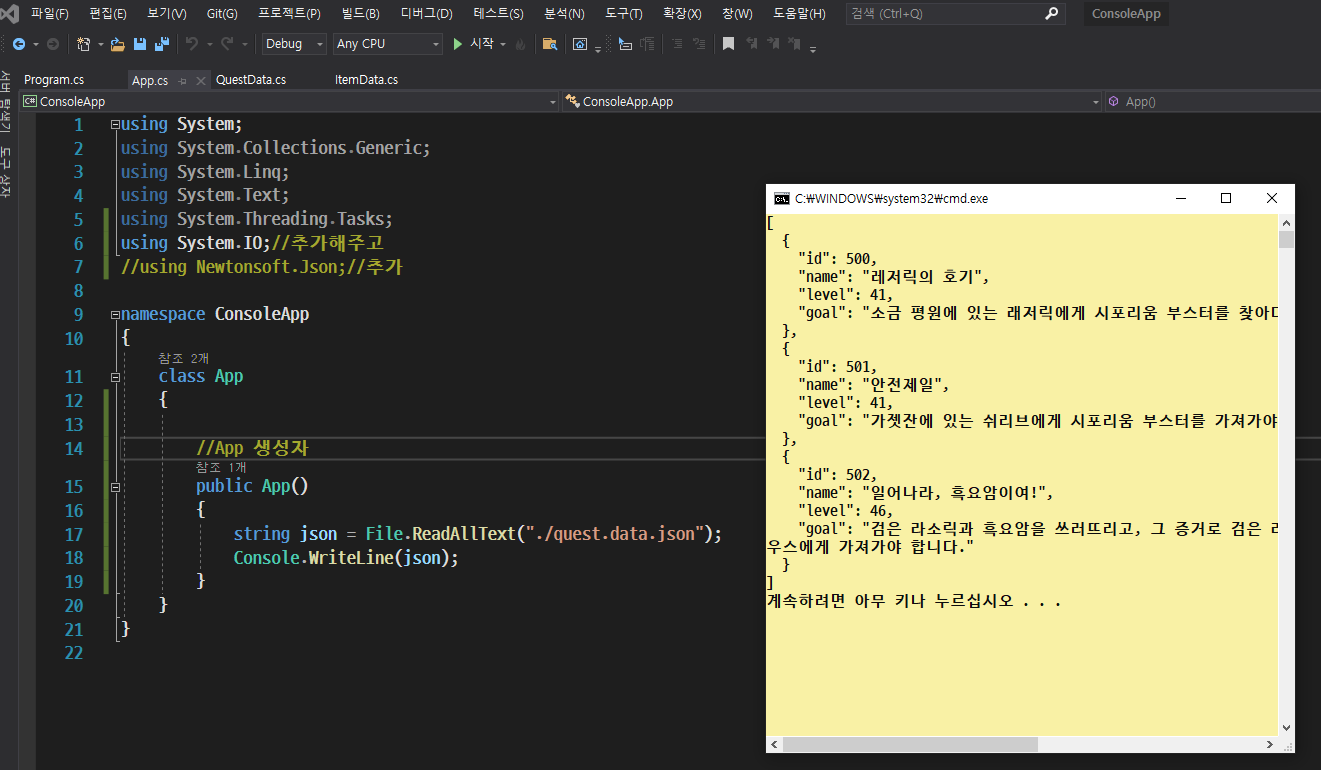
3. 맵핑클래스를 만든다

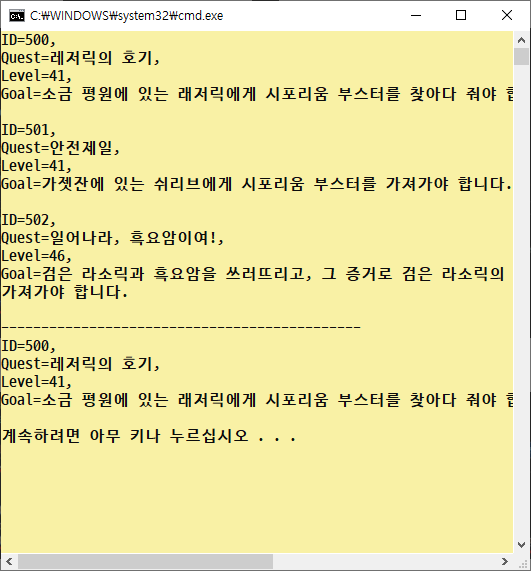
4. 읽어온 json 문자열을 역직렬화 한다
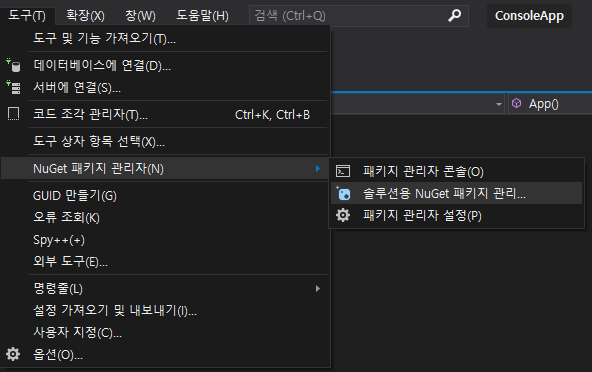
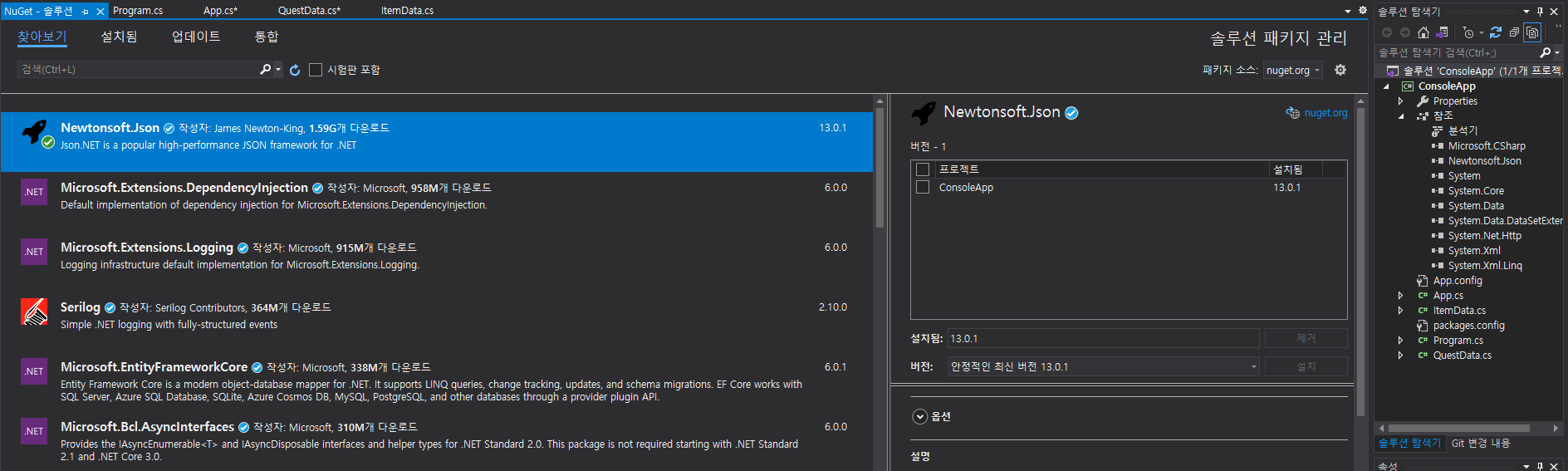

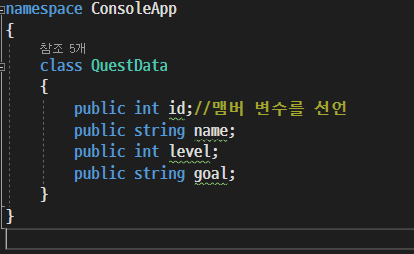
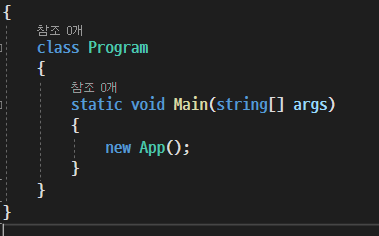
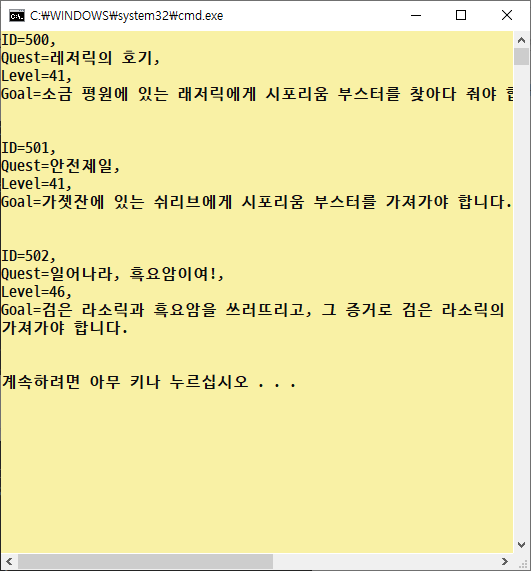
5. 사전에 넣는다
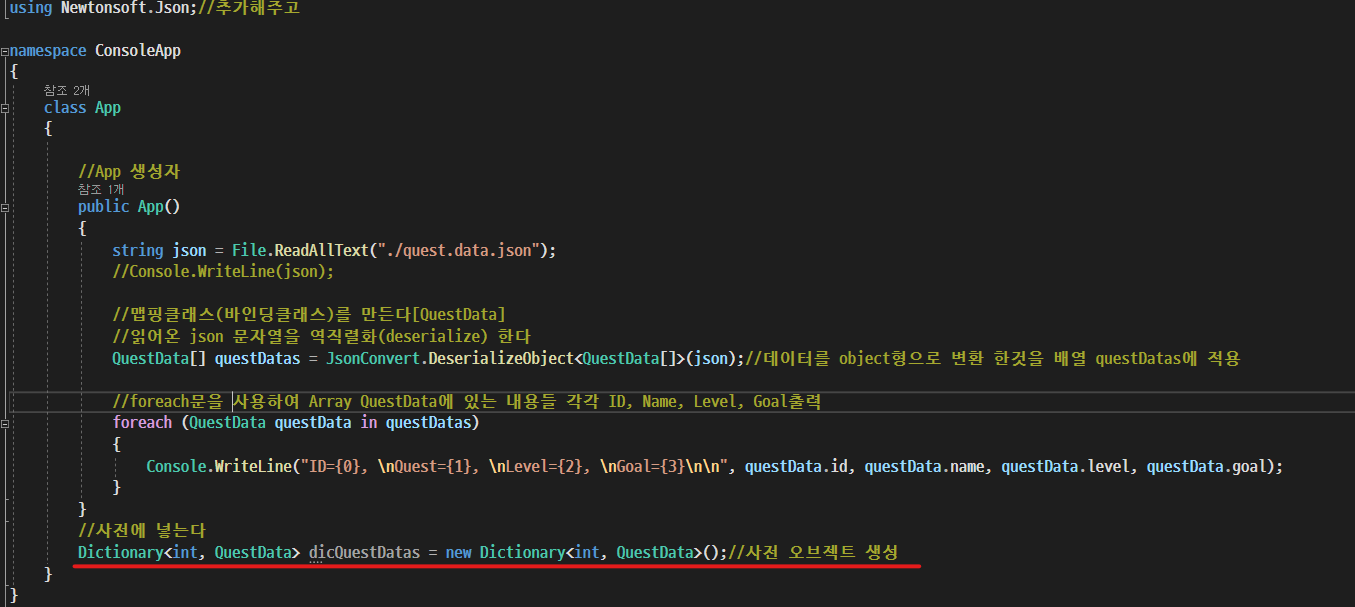
Q2.본인이 작성한 내용을 엑셀에 작성한 후 다음과 같이 작성해본다. (5번)
1. JSON파일을만든다
2. JSON파일을 읽는다
3. 맵핑클래스를 만든다
4. 읽어온 json 문자열을 역직렬화 한다
5. 사전에 넣는다
Q2-1

-----
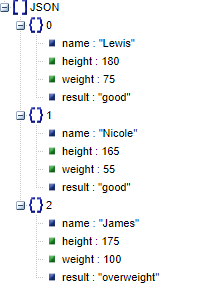
HealtResult
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class HealthResult
{
public string name;
public int height;
public int weight;
public string result;
}
}
App
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;//추가해주고
using Newtonsoft.Json;//추가해주고
namespace ConsoleApp
{
class App
{
//생성자
public App()
{
string json = File.ReadAllText("./HealthResult.data.json");
Console.WriteLine(json);
//json 문자열 역직렬화
HealthResult[] healthResult = JsonConvert.DeserializeObject<HealthResult[]>(json); //json 문자열을 역직렬화 하고나서 배열 healthResult에 대입
foreach(HealthResult results in healthResult)
{
Console.WriteLine("이름:{0}\n 키:{1}\n 몸무게:{2}\n 결과:{3}\n", results.name, results.height, results.weight, results.result);
dicresult.Add(results.name, results);//각 배열 값들을 dictionary에 입력
}
//매핑클래스
HealthResult data = dicresult["Lewis"];
Console.WriteLine("------------------\n이름:{0}\n 키:{1}\n 몸무게:{2}\n 결과:{3}", data.name, data.height, data.weight, data.result);
}
//Dictionary 생성
Dictionary<string, HealthResult> dicresult = new Dictionary<string, HealthResult>();
}
}
Program
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class Program
{
static void Main(string[] args)
{
new App();
}
}
}
Output
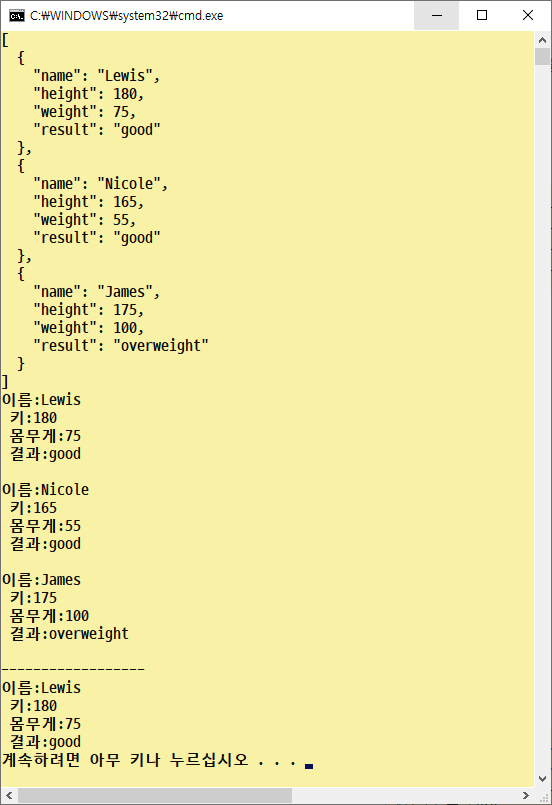
Q2-2

-----
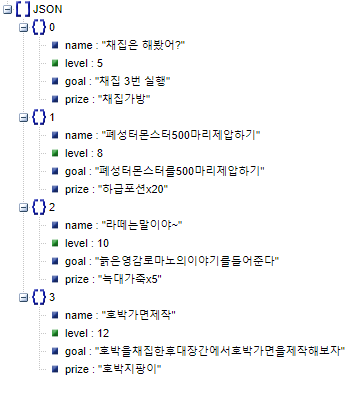
Quest
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class Quest
{
public string name;
public int level;
public string goal;
public string prize;
}
}
App
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;//추가해주고
using Newtonsoft.Json;//추가해주고
namespace ConsoleApp
{
class App
{
//Dictionary 생성
Dictionary<string, Quest> dicQuest = new Dictionary<string, Quest>();
//생성자
public App()
{
//json file 읽기
string json = File.ReadAllText("./Quest.data.json");
//Console.WriteLine(json);
//json 문자열 역직렬화
Quest[] quests = JsonConvert.DeserializeObject<Quest[]>(json);
//딕셔네리에 json object 넣기
foreach(Quest quest in quests)
{
Console.WriteLine("name:{0}\n level:{1}\n goal:{2}\n prize{3}\n", quest.name, quest.level, quest.goal, quest.prize);//이 값들을
dicQuest.Add(quest.name, quest);//딕셔네리에 name, value를 넣었다
}
//매핑클래스 (딕셔네리값을 대입)
Quest data = dicQuest["채집은 해봤어?"];
Console.WriteLine("----------------\n name:{0}\n level:{1}\n goal:{2}\n prize{3}\n", data.name, data.level, data.goal, data.prize);
}
}
}
Program
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class Program
{
static void Main(string[] args)
{
new App();
}
}
}
Output
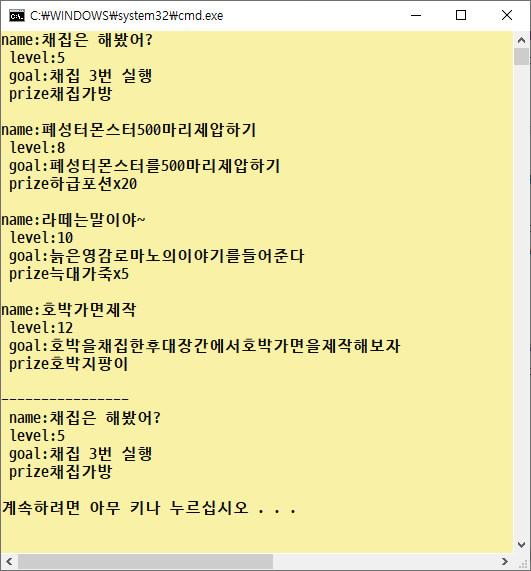
Q2-3
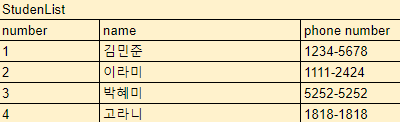
-----
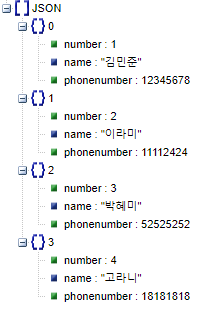
StudentList
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class StudentList
{
public int number;
public string name;
public int phonenumber;
}
}
App
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;//추가해주고
using Newtonsoft.Json;//추가해주고
namespace ConsoleApp
{
class App
{
//Dictionary 생성
Dictionary<int, StudentList> dicList = new Dictionary<int, StudentList>();
//생성자
public App()
{
//json file 읽기
string json = File.ReadAllText("./StudentList.data.json");
//Console.WriteLine(json);
//json 문자열 역직렬화
StudentList[] studentLists = JsonConvert.DeserializeObject<StudentList[]>(json);
//딕셔네리에 json object 넣기
foreach(StudentList list in studentLists)
{
Console.WriteLine($"number:{list.number}\n name:{list.name}\n phone number:{list.phonenumber}");
dicList.Add(list.number, list);
}
//매핑클래스 (딕셔네리값을 대입)
StudentList data = dicList[3];
Console.WriteLine($"-------------------\n number:{data.number}\n name:{data.name}\n phone number:{data.phonenumber}");
}
}
}
Program
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class Program
{
static void Main(string[] args)
{
new App();
}
}
}
Output

Q2-4

-----
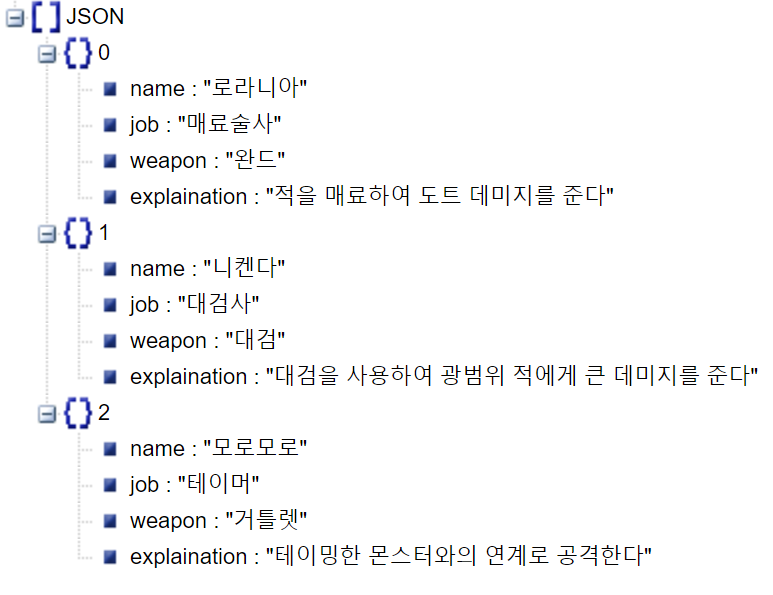
Character
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class Character
{
public string name;
public string job;
public string weapon;
public string explaination;
}
}
App
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;//추가해주고
using Newtonsoft.Json;//추가해주고
namespace ConsoleApp
{
class App
{
Dictionary<string, Character> dicCharacter = new Dictionary<string, Character>();
public App()
{
string json = File.ReadAllText("./Character.data.json");
//Console.WriteLine(json);
Character[] characters = JsonConvert.DeserializeObject<Character[]>(json);
foreach(Character character in characters)
{
Console.WriteLine("name:{0}\n job:{1}\n weapon{2}\n explaination{3}\n", character.name, character.job, character.weapon, character.explaination);
dicCharacter.Add(character.name, character);
}
Character data = dicCharacter["모로모로"];
Console.WriteLine("-----------\n name:{0}\n job:{1}\n weapon{2}\n explaination{3}\n", data.name, data.job, data.weapon, data.explaination);
}
}
}
Program
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class Program
{
static void Main(string[] args)
{
new App();
}
}
}
Output
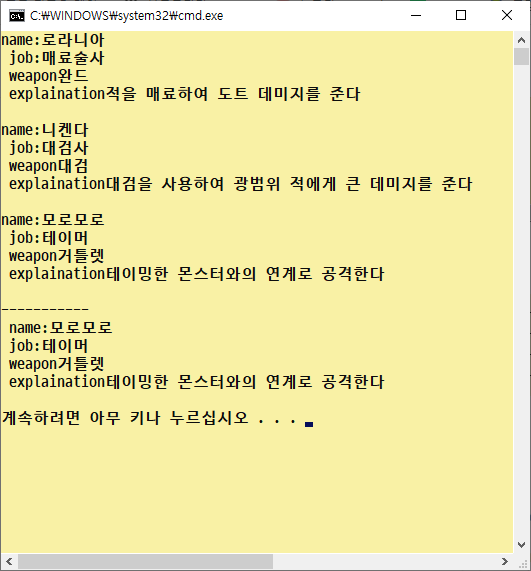
Q2-5

-----
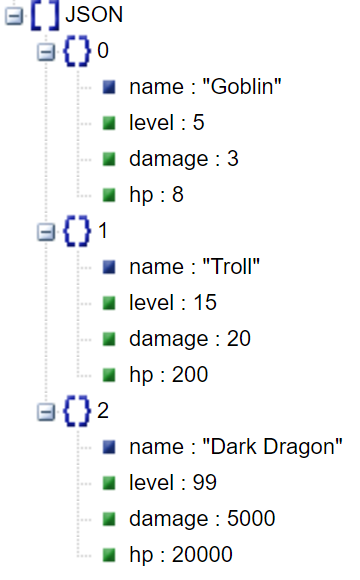
Moster
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class Monster
{
public string name;
public int level;
public int damage;
public int hp;
}
}
App
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;//추가해주고
using Newtonsoft.Json;//추가해주고
namespace ConsoleApp
{
class App
{
Dictionary<string, Monster> dicMonster = new Dictionary<string, Monster>();
//생성자
public App()
{
string json = File.ReadAllText("./Monster.data.json");
//Console.WriteLine(json);
//json file deserialize
Monster[] monsters = JsonConvert.DeserializeObject<Monster[]>(json);
foreach(Monster monster in monsters)
{
Console.WriteLine("name:{0}\n level:{1}\n damage:{2}\n hp:{3}\n", monster.name, monster.level, monster.damage, monster.hp);
dicMonster.Add(monster.name, monster);
}
Monster data = dicMonster["Dark Dragon"];
Console.WriteLine("----------------\n name:{0}\n level:{1}\n damage:{2}\n hp:{3}\n", data.name, data.level, data.damage, data.hp);
}
}
}
Program
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp
{
class Program
{
static void Main(string[] args)
{
new App();
}
}
}
Output
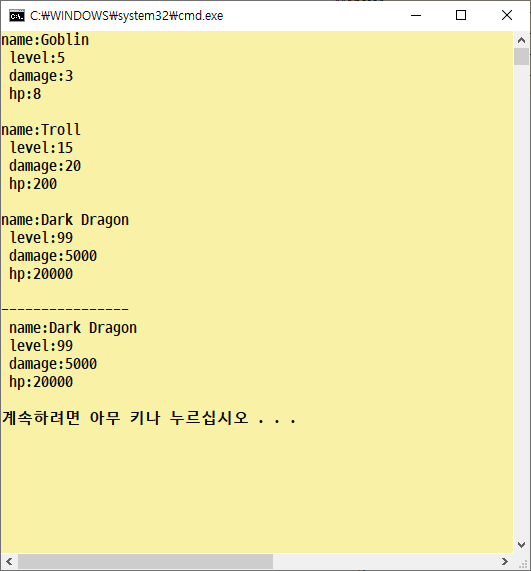
후기:
처음에는 뭐가 뭔지도 모르겠었는데 이제는 입으로 말하면서 타이핑 할 수 있을 정도로 익숙해졌다.
'C# > 수업 내용' 카테고리의 다른 글
1/11 - (테이블만들기, Json변환, 파일읽고쓰기, 직렬화, 역직렬화, 사전넣기, 싱글톤만들기) (0) | 2022.01.12 |
---|---|
1/10 - dictionary2 / 디자인 패턴(싱글톤) (0) | 2022.01.10 |
Dictionary (0) | 2022.01.06 |
Delegate 대리자 2 + Lamda 람다 (0) | 2022.01.05 |
Delegate 대리자1 (0) | 2022.01.04 |